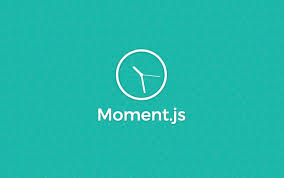
Handling dates and times in JavaScript has always been tricky. The built-in Date
object is unreliable for many use cases, Moment.js was once the de facto standard but has limitations, and various other libraries like date-fns and Luxon have tried to fill the gaps. Now, we have Temporal, a new built-in API designed to replace these outdated approaches.
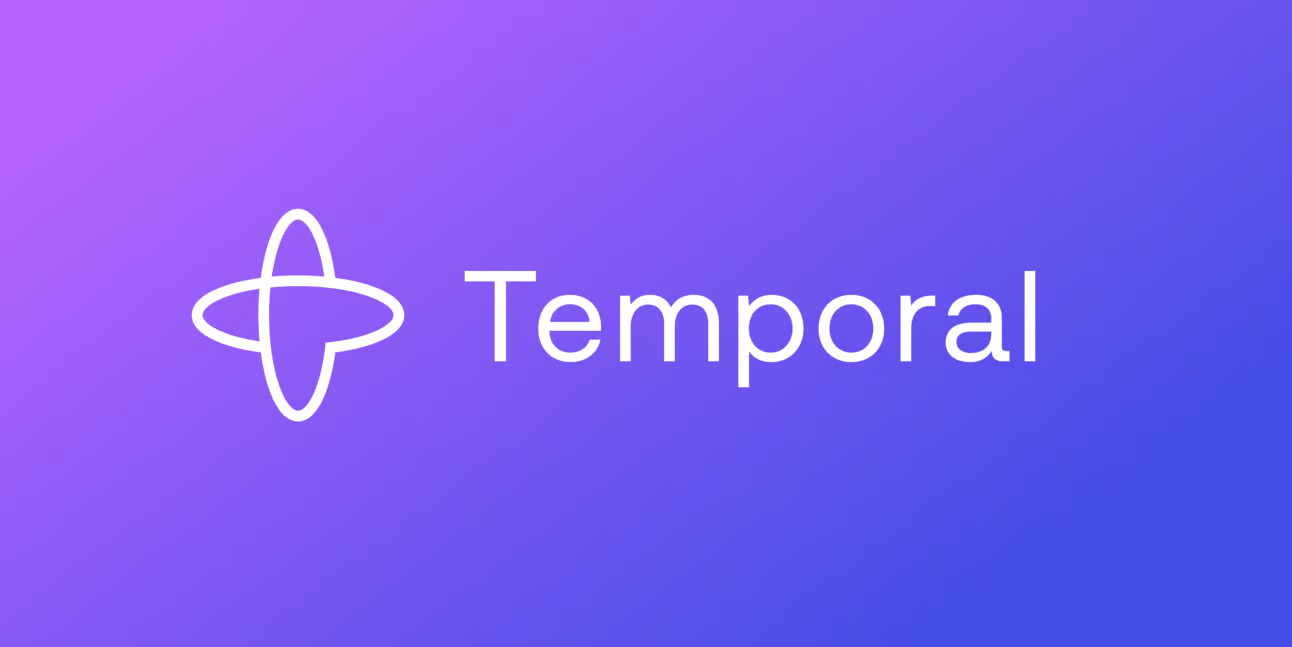
In this blog, we’ll explore why Temporal is the next big thing in JavaScript date handling, compare it to Moment.js, and discuss the trade-offs between Temporal, Moment.js, and the default JavaScript Date
API. Special thanks to CodingWithLewis on YouTube for his insights into this topic, which helped shape this discussion.
Contents
The Problems with JavaScript’s Date
Object
JavaScript’s Date
API has been around since ECMAScript 1 (1997) and hasn’t changed much since. Some major issues include:
- Mutability: The
Date
object is mutable, which can lead to bugs in applications. - Time Zone Limitations:
Date
is always tied to the system’s time zone, making cross-time zone calculations difficult. - Inconsistent Parsing: Different browsers handle date parsing inconsistently.
- Lack of Advanced Features: No built-in support for durations, relative times, or international calendars.
- Millisecond Precision Only: No native support for microseconds or nanoseconds.
To overcome these issues, developers have relied on third-party libraries, most notably Moment.js.
The Rise and Fall of Moment.js
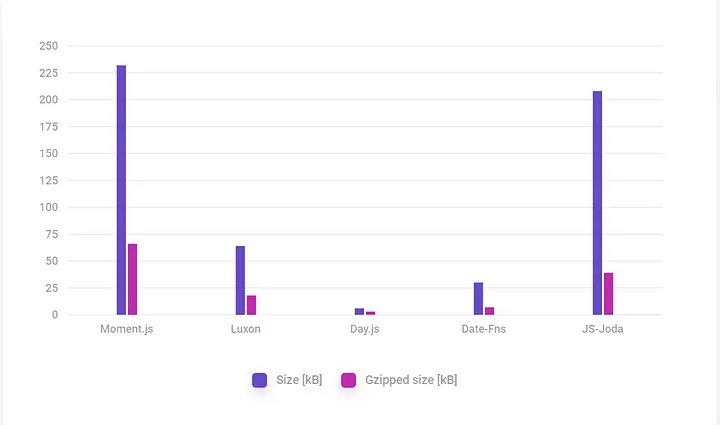
Why Was Moment.js So Popular?
Moment.js emerged as the go-to solution for handling dates in JavaScript. It offered:
- Easy parsing and formatting
- Support for time zones (via
moment-timezone
) - Rich date manipulation methods
- Relative time calculations (
2 days ago
,in 3 hours
)
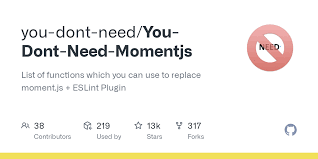
Why Moment.js is No Longer Recommended
Despite its power, Moment.js has major drawbacks:
- Heavy Bundle Size: Moment.js is ~269 KB minified, making it costly for performance-conscious applications.
- Mutability Issues: Dates are mutable by default, leading to unintended side effects.
- Lack of Tree-Shaking: Even if you use only a small part of Moment.js, the whole library is included in your build.
- Better Alternatives Exist: Libraries like date-fns, Luxon, and now Temporal offer better solutions.
Even the Moment.js team deprecated it in favor of newer libraries.
Introducing Temporal: The Future of Date Handling
Temporal is a modern, built-in API that eliminates the shortcomings of both Date
and Moment.js. It provides a consistent, immutable, and powerful way to handle dates and times.
Key Advantages of Temporal
- Immutable Date-Time Objects: No accidental modifications.
- Microsecond Precision: Supports sub-millisecond precision.
- Native Time Zone Support: No need for
moment-timezone
. - Multiple Date & Time Types:
Temporal.Instant
: Exact timestamp (like Unix time).Temporal.PlainDateTime
: Date + time without a time zone.Temporal.ZonedDateTime
: Date + time + time zone.Temporal.Duration
: Represents time intervals.
- Built-in Arithmetic: Easily add/subtract dates without side effects.
- Supports Calendars: Works with non-Gregorian calendars like Islamic and Hebrew.
Example: Using Temporal
// Get the current timestamp (with nanosecond precision)
const now = Temporal.Now.instant();
console.log(now.toString()); // "2025-02-27T14:35:00.123456789Z"
// Create a specific date
const date = Temporal.PlainDate.from('2025-02-27');
console.log(date.toString()); // "2025-02-27"
// Add 10 days
const newDate = date.add({ days: 10 });
console.log(newDate.toString()); // "2025-03-09"
// Convert to a specific time zone
const zonedDateTime = Temporal.Now.zonedDateTimeISO('America/New_York');
console.log(zonedDateTime.toString());
// "2025-02-27T09:35:00.123456789-05:00[America/New_York]"
Comparing Temporal, Moment.js, and the Default Date API
Feature | JavaScript Date | Moment.js | Temporal |
---|---|---|---|
Mutability | Mutable | Mutable | Immutable |
Time Zone Support | Limited | Yes (moment-timezone ) | Yes (native) |
Parsing Consistency | Inconsistent | Good | Excellent |
Performance | Fast but limited | Slow (large bundle) | Fast |
Tree-Shaking | N/A (built-in) | No | Yes (native) |
Microsecond Precision | No (milliseconds only) | No | Yes |
Built-in Arithmetic | Limited | Yes, but can be tricky | Yes, and straightforward |
Bundle Size | N/A | ~269 KB | None (built-in) |
Support for Different Calendars | No | Limited | Yes |
Trade-offs
- Default
Date
API: Lightweight but unreliable and lacks features. - Moment.js: Feature-rich but bloated and mutable.
- Temporal: Modern, powerful, and built-in, but not yet widely supported.
Should You Migrate to Temporal?
Use Temporal If:
✅ You want a modern, immutable API for date handling.
✅ You need precise and reliable date calculations.
✅ Your application involves time zones or durations.
✅ You prefer a zero-dependency approach (Temporal is built-in).
Stick with Moment.js If:
⚠️ Your project is already heavily using Moment.js, and migration would be costly.
⚠️ You don’t mind the bundle size and just need a quick solution.
Consider Alternative Libraries If:
👉 You need a lightweight alternative but Temporal is not available in your environment yet.
👉 date-fns (modular, tree-shakable) or Luxon (Moment.js-like but better) are good choices.
Final Thoughts
Temporal.js is the future of date handling in JavaScript. It solves the long-standing issues with Date
, surpasses Moment.js in reliability and performance, and provides native support for time zones and durations. While it’s still experimental, it’s worth keeping an eye on and considering for new projects.
If you’re building a modern JavaScript application, you should seriously consider Temporal over older libraries like Moment.js. It’s the next big step in JavaScript date handling—and it’s finally here!
Special Thanks
A big shoutout to CodingWithLewis on YouTube for his insightful content on Temporal.js and JavaScript date handling. His explanations helped shape many of the points discussed in this blog. If you want to dive deeper, check out his YouTube channel for more great coding tutorials.
What’s Next?
- Check out the official Temporal proposal.
- Try Temporal polyfills to experiment with it today.
- If you’re still using Moment.js, consider migrating to a modern alternative.
“For more insights and the latest updates, explore our blog archives or visit nomadule.com for more.”